以腾讯企业邮箱为例,讲解如何用 spring-boot 发送邮件
背景
因公司内部平台需要用公家的企业邮箱账号发送邮件给运维人员。
发邮件是一个很常见的功能,代码本身并不复杂,有坑的地方主要在于各家邮件厂家的设置。故此记录下来。
下面以腾讯企业邮箱为例,讲解如何用spring-boot发送邮件。
添加依赖项
1 2 3 4 5
| <!-- SpringBoot Mail --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-mail</artifactId> </dependency>
|
application.yml配置
1 2 3 4 5 6 7 8 9 10 11 12 13
| spring: mail: host: smtp.exmail.qq.com # 邮箱服务器地址 username: xxxx@puscene.com # 这里填写企业邮箱 password: **************** # 这里填写企业邮箱登录密码 properties: mail: smtp: auth: true socketFactory: class: javax.net.ssl.SSLSocketFactory fallback: false port: 465
|
企业邮箱如果未开启安全登录,就不需要授权码了,直接填写登录密码即可。如果开启了安全登录,参考下图:
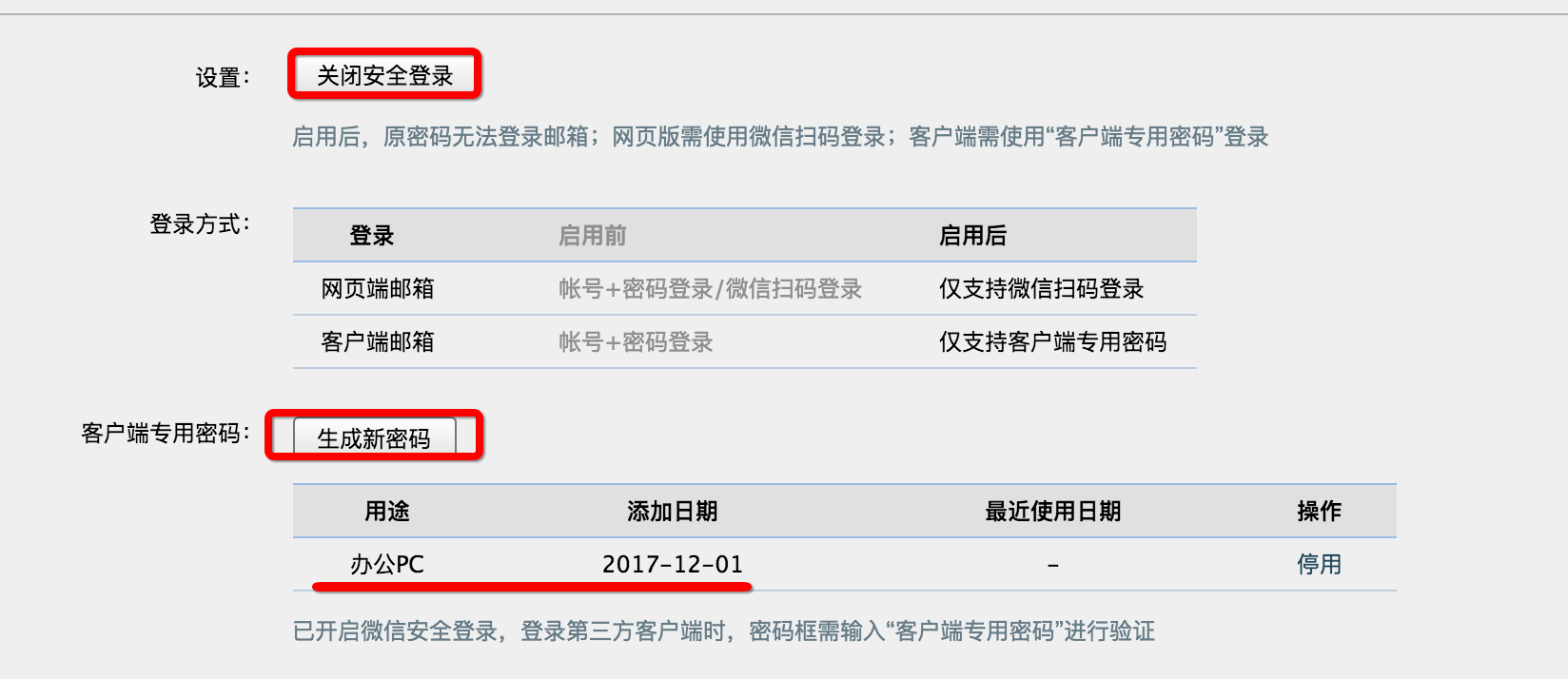
则password这里,需要填写客户端专用密码
发送代码示例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36
| import org.springframework.mail.SimpleMailMessage; import org.springframework.scheduling.annotation.Async; import org.springframework.stereotype.Service; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.beans.factory.annotation.Value; import org.springframework.mail.javamail.JavaMailSender;
@Service public class SendMailUtil {
@Autowired private JavaMailSender mailSender;
@Value("${spring.mail.username}") private String from;
@Async public void sendMail(String title, String url, String email) { SimpleMailMessage message = new SimpleMailMessage(); message.setFrom(from); message.setSubject(title); message.setTo(email); message.setText(url); System.out.println("lihm"); mailSender.send(message); } }
|
注意:
最开始我用单元测试的方式运行发送代码。邮箱未收到邮件。
后面运行 Application 之后再测试才收到邮件
扩展
个人邮箱的发送可以参看Springboot 快速实现邮件发送功能
附上常见邮箱服务器地址:
1 2
| smtp.163.com smtp.qq.com
|
参考链接